파일럿 프로젝트
한장의 사진으로 동영상에 있는 동일인을 찾는 프로젝트를 성공 했습니다.
1. 동영상의 각 프레임에서 사람의 얼굴을 탐지
2. 특정인 인지 결정
Tensorflow 2.8 이용 했습니다.
import cv2
import os
from mtcnn import MTCNN
from deepface import DeepFace
from PIL import Image
import matplotlib.pyplot as plt
# ==========================================
# 1. 동영상 프레임 추출
# ==========================================
def extract_frames(video_path, output_dir):
os.makedirs(output_dir, exist_ok=True)
video = cv2.VideoCapture(video_path)
frame_rate = video.get(cv2.CAP_PROP_FPS) # FPS
success, frame = video.read()
frame_count = 0
while success:
frame_filename = f"{output_dir}/frame_{frame_count:05d}.jpg"
cv2.imwrite(frame_filename, frame)
success, frame = video.read()
frame_count += 1
print(f"총 {frame_count} 프레임 추출 완료.")
return frame_rate, frame_count
# ==========================================
# 2. 사람 얼굴 탐지 (MTCNN)
# ==========================================
def detect_faces(frame_path):
detector = MTCNN()
img_array = cv2.cvtColor(cv2.imread(frame_path), cv2.COLOR_BGR2RGB)
detections = detector.detect_faces(img_array)
return detections
# ==========================================
# 3. 특정인 탐지 (DeepFace)
# ==========================================
def compare_faces(reference_image_path, frame_path, threshold=0.4):
try:
result = DeepFace.verify(
img1_path=reference_image_path,
img2_path=frame_path,
model_name="Facenet",
enforce_detection=False
)
distance = result['distance']
return distance < threshold, distance
except Exception as e:
return False, None
# ==========================================
# 4. 탐지된 타임라인 시각화
# ==========================================
def visualize_timeline(timestamps, video_length):
plt.figure(figsize=(12, 2))
plt.scatter(timestamps, [1] * len(timestamps), marker="|", color="red")
plt.yticks([])
plt.xlabel("Time (seconds)")
plt.title("톰 행크스 등장 타임라인")
plt.xlim(0, video_length)
plt.show()
# ==========================================
# 5. 메인 실행 로직
# ==========================================
def main():
# 입력 경로 설정
video_path = "saving_private_ryan.mp4" # 동영상 파일
reference_image_path = "tomhanks.jpg" # 톰 행크스 사진
output_frames_dir = "./frames" # 프레임 저장 경로
# 1. 동영상에서 프레임 추출
frame_rate, frame_count = extract_frames(video_path, output_frames_dir)
# 2. 프레임마다 얼굴 탐지 및 특정인 탐지
detected_timestamps = []
for frame_file in sorted(os.listdir(output_frames_dir)):
frame_path = os.path.join(output_frames_dir, frame_file)
print(f"프레임 처리 중: {frame_file}")
# 얼굴 탐지
faces = detect_faces(frame_path)
if not faces:
continue
# 특정인(톰 행크스) 탐지
is_match, distance = compare_faces(reference_image_path, frame_path)
if is_match:
frame_number = int(frame_file.split("_")[1].split(".")[0])
timestamp = frame_number / frame_rate
detected_timestamps.append(timestamp)
print(f"> 톰 행크스 등장: 프레임 {frame_number} (시간: {timestamp:.2f}s, 거리: {distance:.2f})")
# 3. 타임라인 시각화
video_length = frame_count / frame_rate # 동영상 길이 (초)
visualize_timeline(detected_timestamps, video_length)
# 4. 탐지된 타임스탬프 출력
print("\n톰 행크스가 탐지된 시간:")
print(detected_timestamps)
# 실행
if __name__ == "__main__":
main()
숫자 필사 인식 프로젝트를 진행 했습니다. MNIST 0~9까지의 필사 6만개를 지도 데이터를 이용해 테스트 데이터의 99%까지 인식 했습니다.
Tensorflow 2.0 이용 했습니다.
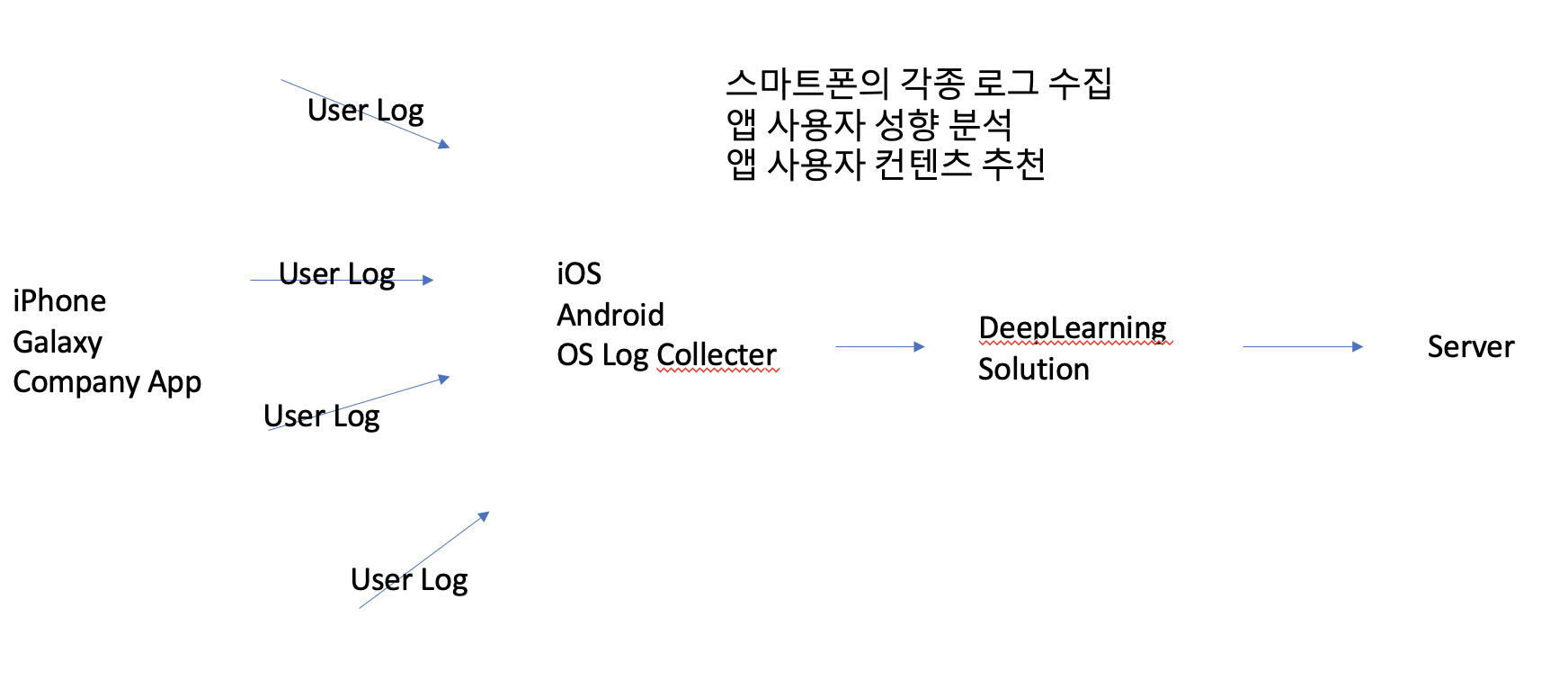
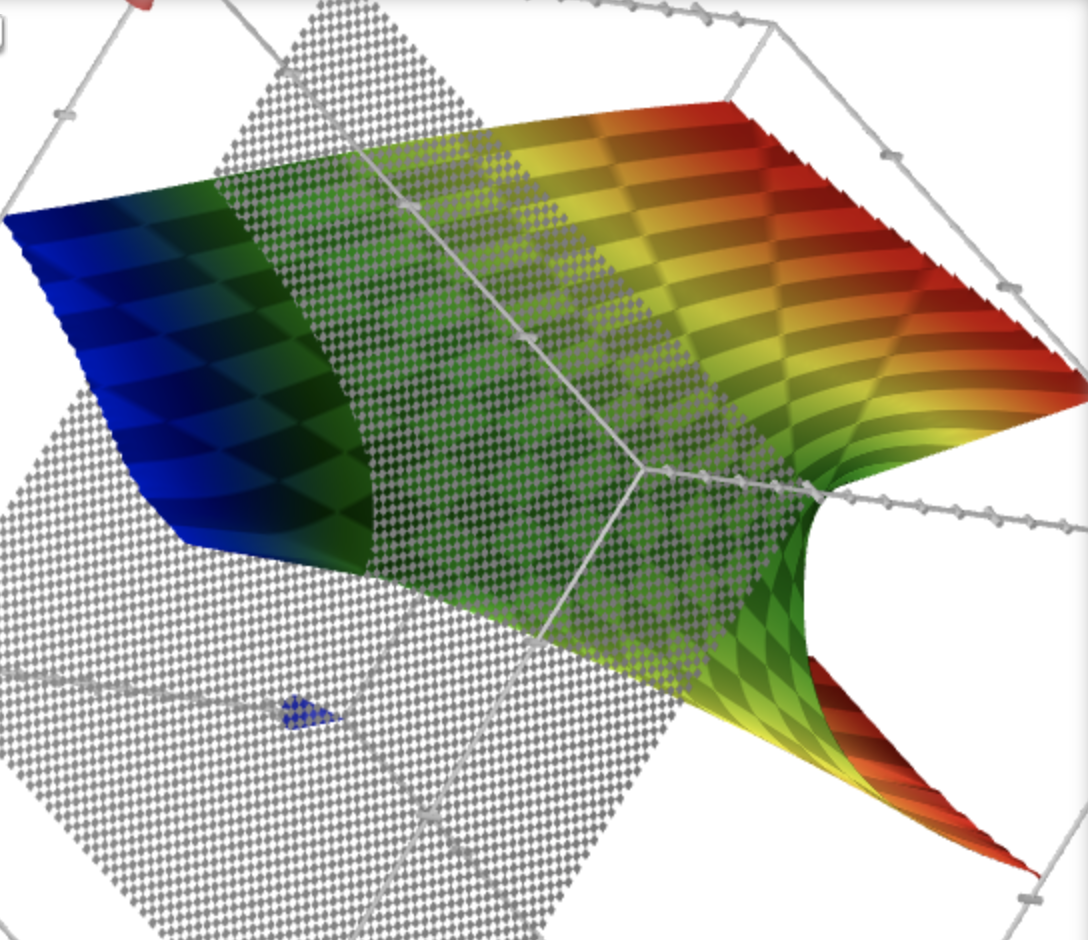